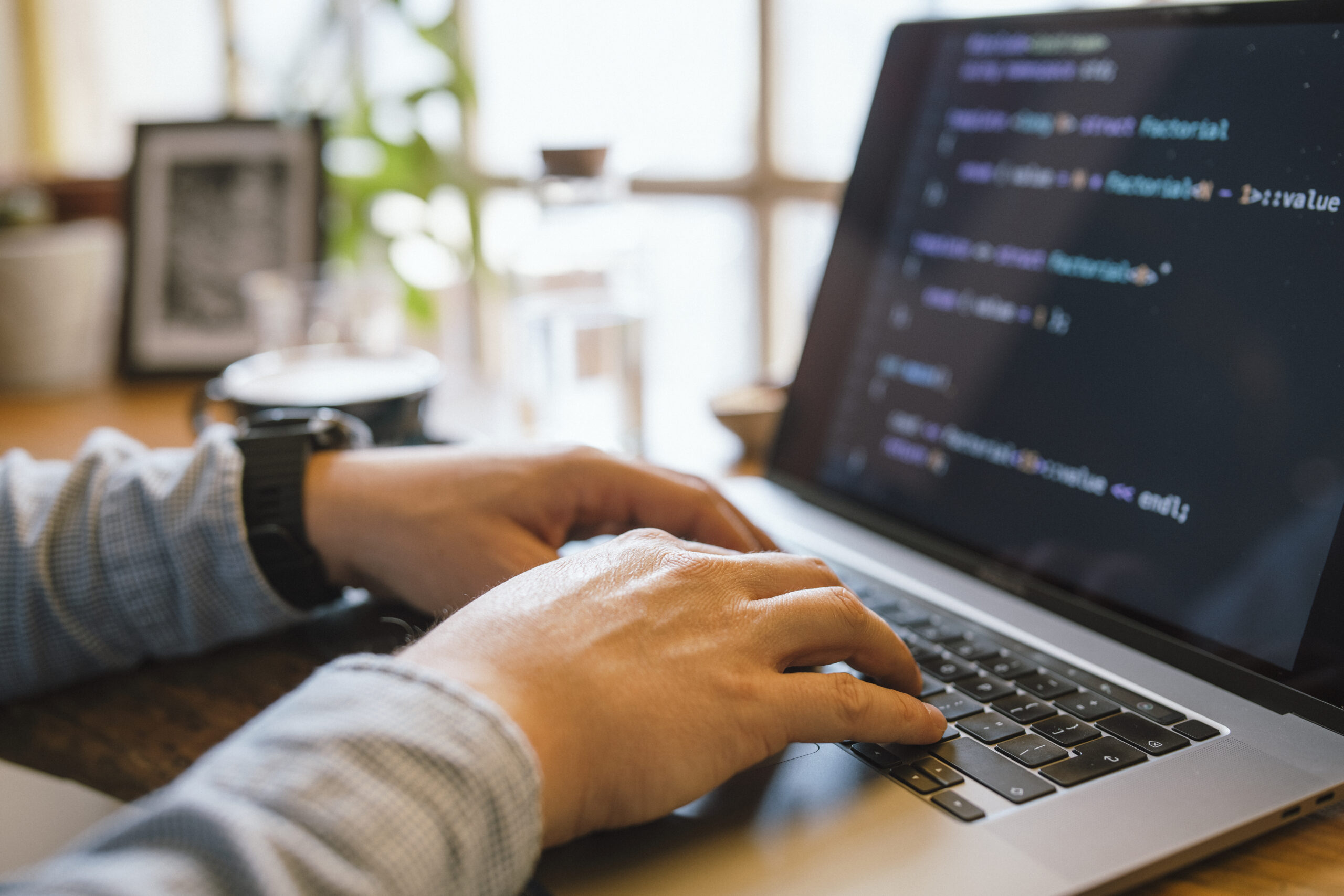
Debugging is Just about the most critical — but typically forgotten — skills inside a developer’s toolkit. It is not almost repairing damaged code; it’s about knowledge how and why points go wrong, and Studying to Feel methodically to resolve difficulties proficiently. No matter if you are a rookie or maybe a seasoned developer, sharpening your debugging techniques can help you save hrs of stress and considerably transform your efficiency. Here's various tactics that will help builders stage up their debugging recreation by me, Gustavo Woltmann.
Master Your Tools
One of the quickest ways builders can elevate their debugging capabilities is by mastering the equipment they use each day. Whilst writing code is a person Component of growth, realizing how you can connect with it proficiently for the duration of execution is equally vital. Modern-day growth environments come Geared up with strong debugging capabilities — but quite a few developers only scratch the area of what these instruments can do.
Acquire, as an example, an Integrated Enhancement Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These instruments let you set breakpoints, inspect the worth of variables at runtime, step as a result of code line by line, and in many cases modify code within the fly. When used the right way, they Enable you to notice just how your code behaves throughout execution, which happens to be invaluable for monitoring down elusive bugs.
Browser developer tools, for instance Chrome DevTools, are indispensable for front-conclude builders. They let you inspect the DOM, check network requests, look at real-time effectiveness metrics, and debug JavaScript within the browser. Mastering the console, resources, and community tabs can change disheartening UI problems into workable duties.
For backend or procedure-degree builders, resources like GDB (GNU Debugger), Valgrind, or LLDB give deep Regulate more than managing processes and memory administration. Learning these instruments may have a steeper Discovering curve but pays off when debugging efficiency issues, memory leaks, or segmentation faults.
Outside of your IDE or debugger, become relaxed with Edition Regulate units like Git to know code historical past, obtain the precise moment bugs had been launched, and isolate problematic improvements.
Finally, mastering your tools indicates going past default options and shortcuts — it’s about establishing an personal familiarity with your progress ecosystem so that when issues arise, you’re not misplaced at midnight. The better you understand your resources, the more time you'll be able to commit fixing the actual issue instead of fumbling via the process.
Reproduce the Problem
Probably the most crucial — and often overlooked — measures in successful debugging is reproducing the trouble. Prior to jumping into your code or making guesses, builders have to have to produce a dependable ecosystem or circumstance the place the bug reliably appears. With out reproducibility, correcting a bug results in being a activity of prospect, generally resulting in wasted time and fragile code changes.
The initial step in reproducing a difficulty is gathering just as much context as is possible. Request questions like: What steps resulted in The difficulty? Which natural environment was it in — advancement, staging, or production? Are there any logs, screenshots, or mistake messages? The more element you have got, the much easier it turns into to isolate the precise situations under which the bug happens.
When you’ve gathered ample info, seek to recreate the challenge in your local setting. This could indicate inputting exactly the same facts, simulating equivalent person interactions, or mimicking method states. If The problem appears intermittently, look at writing automated checks that replicate the edge scenarios or state transitions concerned. These checks not just assistance expose the trouble and also stop regressions Later on.
In some cases, the issue may very well be surroundings-unique — it might come about only on sure operating programs, browsers, or less than particular configurations. Making use of instruments like Digital machines, containerization (e.g., Docker), or cross-browser testing platforms could be instrumental in replicating these bugs.
Reproducing the problem isn’t just a stage — it’s a way of thinking. It necessitates patience, observation, along with a methodical strategy. But as soon as you can continuously recreate the bug, you might be already halfway to fixing it. Having a reproducible situation, You need to use your debugging instruments extra effectively, test possible fixes securely, and talk much more Obviously along with your crew or consumers. It turns an abstract complaint into a concrete obstacle — Which’s where by builders prosper.
Read through and Recognize the Error Messages
Error messages tend to be the most respected clues a developer has when some thing goes wrong. Rather than looking at them as discouraging interruptions, builders must find out to treat mistake messages as immediate communications through the technique. They usually tell you what precisely took place, the place it occurred, and sometimes even why it transpired — if you understand how to interpret them.
Begin by reading the information meticulously and in total. Many builders, particularly when under time force, glance at the first line and promptly commence making assumptions. But further within the mistake stack or logs may possibly lie the accurate root induce. Don’t just copy and paste mistake messages into serps — read through and comprehend them to start with.
Split the mistake down into components. Can it be a syntax error, a runtime exception, or maybe a logic error? Will it stage to a certain file and line quantity? What module or purpose triggered it? These issues can manual your investigation and place you toward the accountable code.
It’s also helpful to grasp the terminology of your programming language or framework you’re employing. Mistake messages in languages like Python, JavaScript, or Java usually observe predictable patterns, and Finding out to acknowledge these can significantly hasten your debugging process.
Some problems are imprecise or generic, As well as in People cases, it’s vital to look at the context wherein the error occurred. Check out similar log entries, input values, and recent improvements in the codebase.
Don’t neglect compiler or linter warnings both. These generally precede larger problems and provide hints about probable bugs.
Finally, error messages usually are not your enemies—they’re your guides. Finding out to interpret them the right way turns chaos into clarity, helping you pinpoint problems more quickly, lessen debugging time, and turn into a additional economical and confident developer.
Use Logging Wisely
Logging is Probably the most effective applications inside of a developer’s debugging toolkit. When utilized successfully, it provides genuine-time insights into how an application behaves, assisting you realize what’s taking place under the hood with no need to pause execution or stage with the code line by line.
A great logging technique starts with knowing what to log and at what level. Common logging levels include DEBUG, Facts, Alert, Mistake, and FATAL. Use DEBUG for comprehensive diagnostic info throughout development, Facts for typical gatherings (like prosperous start off-ups), WARN for potential issues that don’t crack the appliance, ERROR for actual complications, and Deadly if the program can’t carry on.
Avoid flooding your logs with abnormal or irrelevant knowledge. An excessive amount of logging can obscure important messages and decelerate your program. Concentrate on vital gatherings, state changes, enter/output values, and demanding conclusion factors inside your code.
Structure your log messages Obviously and regularly. Involve context, for example timestamps, request IDs, and performance names, so it’s easier to trace troubles in distributed devices or multi-threaded environments. Structured logging (e.g., JSON logs) could make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs Permit you to monitor how variables evolve, what conditions are satisfied, and what branches of logic are executed—all without halting This system. They’re Specially worthwhile in output environments the place stepping through code isn’t attainable.
Additionally, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
In the long run, wise logging is about equilibrium and clarity. Having a properly-thought-out logging method, you may reduce the time it requires to identify challenges, acquire further visibility into your purposes, and improve the All round maintainability and dependability within your code.
Believe Just like a Detective
Debugging is not merely a technological activity—it is a method of investigation. To successfully detect and resolve bugs, builders must method the method similar to a detective solving a mystery. This frame of mind can help stop working complicated troubles into workable pieces and follow clues logically to uncover the root trigger.
Start off by gathering evidence. Think about the signs and symptoms of the situation: error messages, incorrect output, or performance difficulties. Just like a detective surveys a criminal offense scene, obtain as much related information and facts as it is possible to with out leaping to conclusions. Use logs, exam instances, and person experiences to piece together a clear check here image of what’s taking place.
Following, variety hypotheses. Ask your self: What could possibly be triggering this habits? Have any improvements a short while ago been designed to your codebase? Has this challenge transpired just before below equivalent conditions? The purpose is always to slender down prospects and recognize probable culprits.
Then, exam your theories systematically. Seek to recreate the condition in a managed ecosystem. For those who suspect a certain perform or component, isolate it and validate if The problem persists. Like a detective conducting interviews, talk to your code thoughts and Permit the outcomes guide you closer to the reality.
Shell out close notice to little specifics. Bugs normally disguise in the the very least predicted places—similar to a missing semicolon, an off-by-just one error, or simply a race issue. Be thorough and client, resisting the urge to patch the issue devoid of totally knowledge it. Short-term fixes may perhaps disguise the true dilemma, only for it to resurface later.
Lastly, retain notes on Everything you tried out and learned. Equally as detectives log their investigations, documenting your debugging procedure can help save time for long term difficulties and enable Other individuals comprehend your reasoning.
By wondering similar to a detective, developers can sharpen their analytical techniques, method troubles methodically, and become simpler at uncovering hidden challenges in sophisticated units.
Publish Exams
Producing tests is one of the best tips on how to boost your debugging techniques and overall improvement efficiency. Exams not merely enable catch bugs early but additionally serve as a security Web that offers you assurance when creating adjustments to the codebase. A perfectly-tested application is easier to debug because it enables you to pinpoint specifically in which and when a difficulty takes place.
Begin with device exams, which target specific features or modules. These modest, isolated assessments can speedily reveal no matter whether a particular piece of logic is Operating as anticipated. Whenever a check fails, you instantly know exactly where to appear, significantly lessening some time expended debugging. Unit tests are especially practical for catching regression bugs—difficulties that reappear soon after Formerly being fastened.
Up coming, integrate integration checks and conclude-to-stop tests into your workflow. These assistance be certain that different parts of your software perform together effortlessly. They’re notably helpful for catching bugs that manifest in advanced techniques with numerous factors or companies interacting. If some thing breaks, your checks can let you know which A part of the pipeline unsuccessful and below what disorders.
Composing tests also forces you to definitely think critically regarding your code. To check a characteristic properly, you may need to know its inputs, predicted outputs, and edge instances. This standard of comprehending Obviously leads to higher code structure and less bugs.
When debugging a concern, writing a failing examination that reproduces the bug is usually a strong starting point. Once the examination fails continuously, you are able to center on correcting the bug and view your take a look at go when the issue is solved. This approach makes sure that a similar bug doesn’t return in the future.
In a nutshell, crafting tests turns debugging from a discouraging guessing activity into a structured and predictable course of action—encouraging you catch far more bugs, speedier and much more reliably.
Just take Breaks
When debugging a tough difficulty, it’s easy to become immersed in the issue—watching your display screen for hrs, hoping Alternative after Answer. But Just about the most underrated debugging equipment is actually stepping absent. Getting breaks will help you reset your head, lower annoyance, and infrequently see The difficulty from the new point of view.
When you are way too near to the code for also prolonged, cognitive tiredness sets in. You could commence overlooking apparent mistakes or misreading code which you wrote just hours earlier. In this point out, your Mind gets considerably less productive at issue-solving. A brief wander, a espresso crack, or maybe switching to another undertaking for ten–15 minutes can refresh your emphasis. Several developers report locating the root of a dilemma when they've taken time and energy to disconnect, allowing their subconscious get the job done while in the track record.
Breaks also assist reduce burnout, Primarily through more time debugging sessions. Sitting down in front of a monitor, mentally caught, is not only unproductive but will also draining. Stepping away enables you to return with renewed Electrical power and also a clearer attitude. You might quickly recognize a lacking semicolon, a logic flaw, or possibly a misplaced variable that eluded you prior to.
When you’re stuck, a very good guideline is to established a timer—debug actively for forty five–60 minutes, then have a 5–ten moment split. Use that point to move all over, stretch, or do a thing unrelated to code. It may come to feel counterintuitive, Particularly underneath tight deadlines, nonetheless it actually contributes to faster and simpler debugging Ultimately.
In brief, getting breaks is not a sign of weakness—it’s a wise system. It gives your brain House to breathe, improves your viewpoint, and helps you stay away from the tunnel eyesight that often blocks your progress. Debugging can be a psychological puzzle, and relaxation is a component of fixing it.
Master From Every Bug
Every single bug you come upon is more than just A brief setback—It can be an opportunity to expand to be a developer. Whether or not it’s a syntax error, a logic flaw, or maybe a deep architectural difficulty, each one can teach you one thing worthwhile when you take the time to reflect and evaluate what went Improper.
Start off by inquiring on your own a handful of key questions once the bug is resolved: What brought on it? Why did it go unnoticed? Could it have already been caught previously with far better procedures like unit screening, code evaluations, or logging? The solutions usually reveal blind spots inside your workflow or comprehending and enable you to Construct more powerful coding routines shifting forward.
Documenting bugs will also be a wonderful pattern. Retain a developer journal or retain a log in which you Observe down bugs you’ve encountered, how you solved them, and Everything you discovered. Over time, you’ll begin to see styles—recurring troubles or frequent errors—that you could proactively avoid.
In workforce environments, sharing That which you've uncovered from a bug together with your friends might be Specifically potent. Whether it’s via a Slack concept, a brief produce-up, or a quick awareness-sharing session, supporting Other individuals steer clear of the very same concern boosts workforce effectiveness and cultivates a stronger Mastering tradition.
Extra importantly, viewing bugs as lessons shifts your mindset from stress to curiosity. Rather than dreading bugs, you’ll get started appreciating them as crucial parts of your growth journey. In the end, many of the greatest builders aren't those who write best code, but individuals who continuously understand from their mistakes.
Ultimately, Each individual bug you resolve provides a new layer to the talent set. So following time you squash a bug, have a moment to mirror—you’ll occur away a smarter, a lot more able developer because of it.
Conclusion
Increasing your debugging skills will take time, exercise, and patience — however the payoff is big. It will make you a more effective, self-confident, and able developer. The next time you're knee-deep within a mysterious bug, try to remember: debugging isn’t a chore — it’s an opportunity to become far better at Whatever you do.