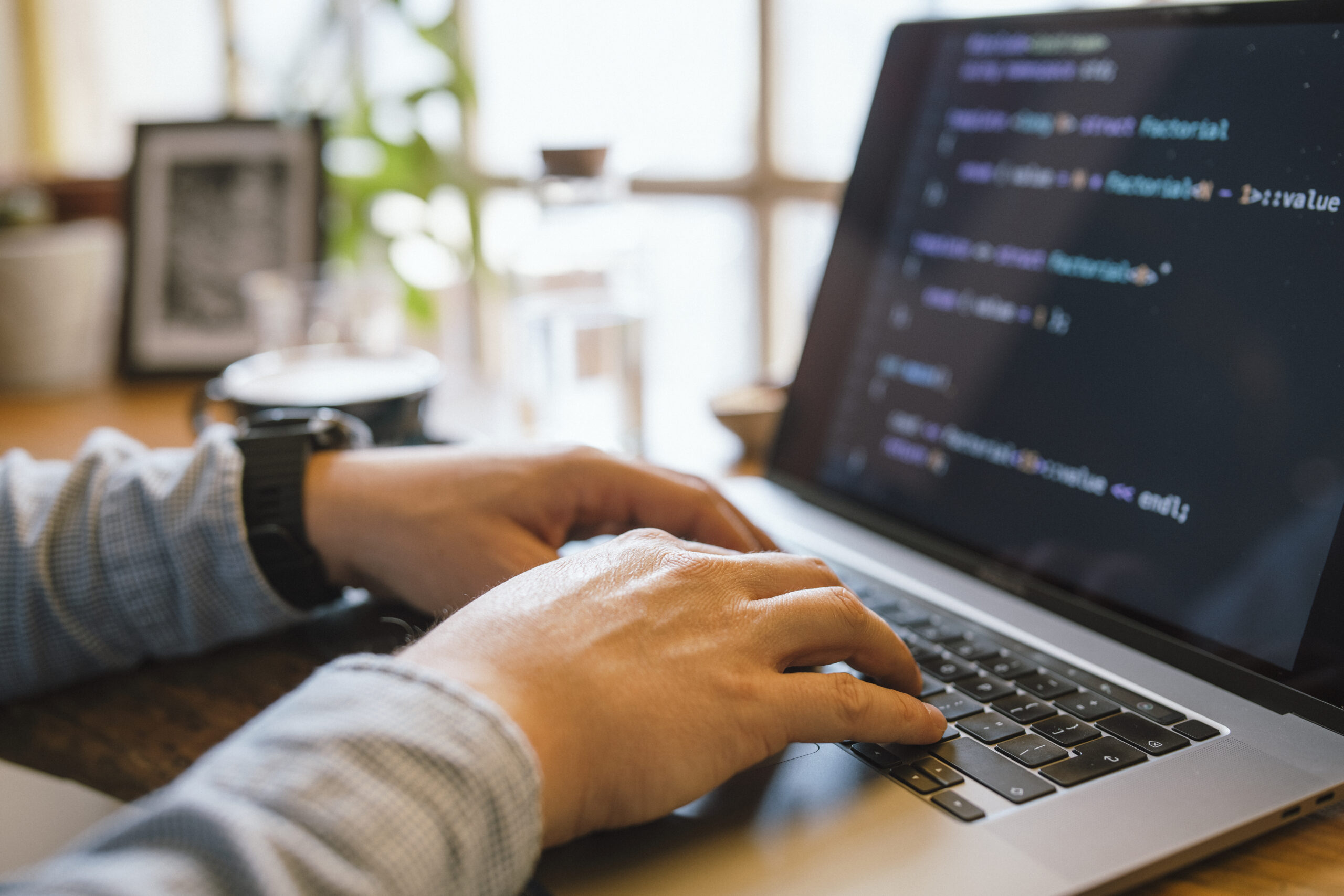
Debugging is one of the most crucial — nonetheless often ignored — capabilities within a developer’s toolkit. It is not almost repairing damaged code; it’s about knowledge how and why points go Completely wrong, and learning to think methodically to solve problems efficiently. Whether or not you're a beginner or perhaps a seasoned developer, sharpening your debugging abilities can preserve hrs of disappointment and drastically boost your productivity. Listed here are various approaches to help you developers stage up their debugging recreation by me, Gustavo Woltmann.
Master Your Equipment
One of many quickest means builders can elevate their debugging capabilities is by mastering the resources they use each day. Whilst writing code is a person Component of growth, realizing how to interact with it correctly through execution is equally important. Modern-day advancement environments arrive equipped with highly effective debugging abilities — but several builders only scratch the floor of what these instruments can do.
Choose, by way of example, an Integrated Progress Surroundings (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These applications enable you to established breakpoints, inspect the value of variables at runtime, move by way of code line by line, as well as modify code on the fly. When utilised properly, they Enable you to observe just how your code behaves during execution, that's priceless for monitoring down elusive bugs.
Browser developer resources, such as Chrome DevTools, are indispensable for front-close developers. They allow you to inspect the DOM, keep an eye on community requests, see authentic-time overall performance metrics, and debug JavaScript while in the browser. Mastering the console, resources, and community tabs can transform aggravating UI difficulties into workable duties.
For backend or technique-level developers, instruments like GDB (GNU Debugger), Valgrind, or LLDB offer deep Handle about running processes and memory administration. Learning these resources might have a steeper Finding out curve but pays off when debugging general performance problems, memory leaks, or segmentation faults.
Over and above your IDE or debugger, turn out to be cozy with Model Command systems like Git to comprehend code historical past, find the exact moment bugs had been launched, and isolate problematic alterations.
In the long run, mastering your applications implies heading over and above default options and shortcuts — it’s about establishing an personal familiarity with your enhancement setting to make sure that when challenges crop up, you’re not shed in the dark. The better you realize your instruments, the greater time it is possible to commit fixing the actual difficulty rather then fumbling as a result of the procedure.
Reproduce the condition
One of the more important — and sometimes neglected — ways in helpful debugging is reproducing the condition. Before leaping in the code or generating guesses, developers need to have to make a constant surroundings or situation exactly where the bug reliably seems. With no reproducibility, correcting a bug gets a recreation of chance, generally resulting in wasted time and fragile code improvements.
The initial step in reproducing a difficulty is gathering just as much context as is possible. Request questions like: What steps triggered The problem? Which atmosphere was it in — enhancement, staging, or generation? Are there any logs, screenshots, or error messages? The greater detail you've got, the easier it will become to isolate the precise circumstances less than which the bug happens.
Once you’ve gathered ample information, endeavor to recreate the issue in your neighborhood atmosphere. This may imply inputting exactly the same facts, simulating equivalent person interactions, or mimicking program states. If The difficulty appears intermittently, look at producing automated exams that replicate the sting instances or point out transitions involved. These exams not just enable expose the condition but also avert regressions Down the road.
At times, The problem may be natural environment-specific — it would transpire only on certain working programs, browsers, or less than distinct configurations. Applying tools like virtual devices, containerization (e.g., Docker), or cross-browser tests platforms could be instrumental in replicating these bugs.
Reproducing the problem isn’t simply a step — it’s a attitude. It involves tolerance, observation, along with a methodical strategy. But when you finally can regularly recreate the bug, you happen to be presently halfway to repairing it. That has a reproducible state of affairs, You should use your debugging equipment more successfully, check prospective fixes safely and securely, and talk far more Plainly with your team or users. It turns an abstract criticism right into a concrete obstacle — Which’s wherever builders prosper.
Examine and Fully grasp the Mistake Messages
Error messages are frequently the most precious clues a developer has when one thing goes Erroneous. Rather than looking at them as disheartening interruptions, builders must find out to treat error messages as direct communications through the program. They frequently tell you what precisely took place, the place it occurred, and occasionally even why it happened — if you know the way to interpret them.
Start off by studying the information thoroughly and in total. Numerous builders, particularly when under time force, glance at the first line and promptly get started earning assumptions. But further within the mistake stack or logs could lie the true root induce. Don’t just copy and paste error messages into search engines — examine and comprehend them to start with.
Split the error down into pieces. Could it be a syntax mistake, a runtime exception, or perhaps a logic mistake? Does it place to a particular file and line selection? What module or perform brought on it? These queries can manual your investigation and place you toward the accountable code.
It’s also practical to comprehend the terminology with the programming language or framework you’re utilizing. Mistake messages in languages like Python, JavaScript, or Java usually comply with predictable patterns, and Finding out to acknowledge these can significantly hasten your debugging procedure.
Some problems are obscure or generic, As well as in Those people instances, it’s important to look at the context by which the error happened. Check linked log entries, enter values, and recent alterations from the codebase.
Don’t overlook compiler or linter warnings either. These typically precede larger sized challenges and provide hints about likely bugs.
Finally, error messages will not be your enemies—they’re your guides. Learning to interpret them appropriately turns chaos into clarity, supporting you pinpoint challenges a lot quicker, reduce debugging time, and become a much more productive and self-confident developer.
Use Logging Sensibly
Logging is Probably the most effective applications in a developer’s debugging toolkit. When used successfully, it provides actual-time insights into how an application behaves, supporting you recognize what’s occurring underneath the hood without needing to pause execution or step through the code line by line.
A great logging technique begins with being aware of what to log and at what stage. Prevalent logging ranges consist of DEBUG, Information, WARN, ERROR, and FATAL. Use DEBUG for detailed diagnostic information during advancement, Details for normal gatherings (like prosperous start out-ups), Alert for opportunity challenges that don’t crack the application, ERROR for precise challenges, and Deadly once the system can’t continue.
Prevent flooding your logs with abnormal or irrelevant facts. An excessive amount logging can obscure crucial messages and slow down your procedure. Center on critical functions, state variations, enter/output values, and significant choice details in the code.
Format your log messages Evidently and continually. Consist of context, which include timestamps, ask for IDs, and performance names, so it’s simpler to trace challenges in distributed systems or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
Through debugging, logs let you observe how variables evolve, what problems are met, and what branches of logic are executed—all devoid of halting the program. They’re Specially valuable in generation environments where stepping by way of code isn’t possible.
On top of that, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
Eventually, intelligent logging is about stability and clarity. That has a well-imagined-out logging solution, you may reduce the time it requires to identify issues, obtain further visibility into your purposes, and improve the All round maintainability and reliability of your respective code.
Imagine Like a Detective
Debugging is not only a complex endeavor—it's a type of investigation. To properly detect and fix bugs, builders ought to solution the process like a detective solving a thriller. This frame of mind can help stop working complex troubles into workable sections and abide by clues logically to uncover the foundation cause.
Begin by gathering evidence. Look at the signs of the condition: mistake messages, incorrect output, or general performance issues. Just like a detective surveys a crime scene, gather as much related info as it is possible to without jumping to conclusions. Use logs, exam conditions, and person experiences to piece alongside one another a transparent photo of what’s taking place.
Up coming, sort hypotheses. Question by yourself: What may be leading to this conduct? Have any modifications recently been made into the codebase? Has this challenge happened in advance of beneath equivalent circumstances? The goal should be to slim down prospects and identify opportunity culprits.
Then, take a look at your theories systematically. Endeavor to recreate the challenge within a controlled natural environment. Should you suspect a specific functionality or element, isolate it and verify if The difficulty persists. Similar to a detective conducting interviews, inquire your code questions and Enable the outcome lead you nearer to the truth.
Fork out close notice to smaller facts. Bugs usually hide from the least envisioned areas—similar to a missing semicolon, an off-by-a person error, or a race condition. Be extensive and affected person, resisting the urge to patch The difficulty with out thoroughly comprehending it. Momentary fixes might cover the real dilemma, only for it to resurface afterwards.
Finally, retain notes on Everything you tried out and learned. Just as detectives log their investigations, documenting your debugging course of action can help you save time for potential difficulties and help Many others comprehend your reasoning.
By contemplating similar to a detective, developers can sharpen their analytical expertise, tactic complications methodically, and turn out to be simpler at uncovering concealed issues in advanced units.
Create Exams
Producing checks is among the simplest methods to boost your debugging capabilities check here and In general development effectiveness. Assessments not simply enable capture bugs early but will also function a security Web that offers you confidence when creating modifications on your codebase. A perfectly-analyzed software is much easier to debug mainly because it helps you to pinpoint exactly in which and when a difficulty happens.
Begin with unit assessments, which center on particular person features or modules. These modest, isolated assessments can speedily reveal regardless of whether a particular piece of logic is working as envisioned. Every time a examination fails, you quickly know in which to appear, considerably decreasing some time used debugging. Device exams are Particularly useful for catching regression bugs—problems that reappear after Beforehand staying mounted.
Subsequent, combine integration assessments and stop-to-end checks into your workflow. These support make certain that various elements of your software operate with each other effortlessly. They’re notably helpful for catching bugs that occur in complex devices with numerous factors or companies interacting. If some thing breaks, your tests can inform you which A part of the pipeline unsuccessful and below what disorders.
Composing checks also forces you to Imagine critically regarding your code. To check a attribute properly, you would like to grasp its inputs, expected outputs, and edge circumstances. This volume of comprehension naturally qualified prospects to raised code construction and less bugs.
When debugging an issue, composing a failing exam that reproduces the bug is often a powerful initial step. When the test fails persistently, you could give attention to repairing the bug and check out your check move when The difficulty is resolved. This strategy ensures that the identical bug doesn’t return Sooner or later.
To put it briefly, writing exams turns debugging from the discouraging guessing video game right into a structured and predictable procedure—supporting you capture extra bugs, quicker plus more reliably.
Take Breaks
When debugging a tricky situation, it’s uncomplicated to be immersed in the problem—staring at your display for hours, making an attempt Resolution immediately after Alternative. But one of the most underrated debugging equipment is actually stepping absent. Getting breaks will help you reset your head, cut down irritation, and infrequently see The difficulty from the new standpoint.
If you're far too near the code for too lengthy, cognitive fatigue sets in. You may begin overlooking obvious errors or misreading code that you wrote just hours before. During this point out, your Mind turns into significantly less effective at dilemma-fixing. A short wander, a espresso split, and even switching to a special task for 10–15 minutes can refresh your aim. Quite a few builders report locating the root of a dilemma after they've taken the perfect time to disconnect, allowing their subconscious perform within the background.
Breaks also enable avert burnout, Specifically throughout for a longer period debugging periods. Sitting before a display, mentally stuck, is not only unproductive but will also draining. Stepping away enables you to return with renewed Electrical power plus a clearer state of mind. You might out of the blue discover a lacking semicolon, a logic flaw, or even a misplaced variable that eluded you before.
When you’re stuck, a fantastic rule of thumb would be to established a timer—debug actively for 45–sixty minutes, then have a 5–ten minute split. Use that time to move all-around, stretch, or do a little something unrelated to code. It might experience counterintuitive, Specifically less than restricted deadlines, however it in fact leads to more rapidly and more effective debugging Over time.
To put it briefly, using breaks is not a sign of weak point—it’s a sensible method. It offers your Mind Area to breathe, enhances your perspective, and will help you steer clear of the tunnel vision that often blocks your development. Debugging is usually a mental puzzle, and rest is a component of resolving it.
Learn From Each and every Bug
Just about every bug you encounter is more than just A brief setback—It is a chance to improve to be a developer. Regardless of whether it’s a syntax mistake, a logic flaw, or maybe a deep architectural difficulty, each one can teach you one thing worthwhile for those who take the time to reflect and evaluate what went Improper.
Start off by inquiring yourself a couple of crucial inquiries when the bug is fixed: What prompted it? Why did it go unnoticed? Could it have been caught earlier with better practices like unit tests, code reviews, or logging? The responses often expose blind places as part of your workflow or comprehending and enable you to Construct more powerful coding routines shifting forward.
Documenting bugs will also be a wonderful pattern. Maintain a developer journal or preserve a log where you Take note down bugs you’ve encountered, the way you solved them, and That which you uncovered. With time, you’ll start to see styles—recurring problems or typical mistakes—you could proactively stay clear of.
In staff environments, sharing Whatever you've discovered from the bug with the peers may be especially highly effective. No matter whether it’s by way of a Slack information, a brief create-up, or A fast expertise-sharing session, supporting Other individuals avoid the similar concern boosts team effectiveness and cultivates a stronger Discovering tradition.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as necessary elements of your enhancement journey. All things considered, some of the finest developers are not the ones who generate excellent code, but individuals that continually master from their blunders.
Eventually, Each and every bug you deal with adds a whole new layer to your ability established. So next time you squash a bug, take a minute to replicate—you’ll come absent a smarter, much more capable developer thanks to it.
Conclusion
Strengthening your debugging competencies requires time, follow, and tolerance — however the payoff is large. It tends to make you a more successful, self-assured, and capable developer. The following time you happen to be knee-deep in a very mysterious bug, remember: debugging isn’t a chore — it’s an opportunity to become superior at Everything you do.